How to Seamlessly Build a React App with a Java Backend
Struggling with React and Java integration? This guide covers setting up your development environment, building a secure Java backend, and integrating APIs with React. Master the process with practical steps. Start now!
Hey there, Enthusiastic developer! 👋
Ready to dive into the exciting world of building React applications with Java backends? If you're looking to create cutting-edge web apps that are both dynamic and robust, you're in the right place. This comprehensive guide will take you from zero to hero in React and Java backend development.
In the age of digital innovation, the demand for dynamic web applications is ever-increasing. React, a JavaScript library for building user interfaces, has gained immense popularity for its flexibility and efficiency. When paired with a Java backend, known for its scalability and reliability, you can create web applications that truly stand out.
In this guide, we'll cover everything you need to know about building React apps with Java backends. From setting up your development environment to deploying your application, we've got you covered. Let's embark on this journey of discovery and development. 🚀
Understanding the Basics of React and Java
Let's start with the fundamentals. React is a JavaScript library for building user interfaces, primarily maintained by Facebook and a community of individual developers and companies.
Java, on the other hand, is a versatile and powerful programming language used for building server-side applications. Combining React's front-end capabilities with Java's back-end robustness unlocks a world of possibilities.

Setting Up Your Development Environment
To begin this journey, you need the right tools and environment. Let's get your development environment up and running for React and Java backend development. Don't worry; we'll guide you step by step.
For React Development
For Java Backend Development
Building the Java Backend
Building the Java backend is the foundation of your project. It's where you'll create the logic, manage data, and handle requests from your React front end. Let's explore the essential components.
Choosing the Right Java Framework
Spring Boot
Known for its simplicity and rapid development, Spring Boot is an excellent choice for building RESTful APIs. It provides a wide range of frameworks and tools to enhance productivity and performance:
- Spring MVC: Simplifies the development of web applications with a robust model-view-controller architecture.
- Spring Data JPA: Eases the implementation of data access layers by using JPA (Java Persistence API) to interact with databases.
- Spring Security: Provides comprehensive security services for Java EE-based enterprise software applications.
- Spring Cloud: Facilitates the development of distributed systems and microservices with cloud-native features.
- Spring Batch: Designed for processing large volumes of data, it supports batch processing essential for enterprise-level applications.
- Micronaut: A modern, JVM-based framework designed for building modular, easily testable microservice and serverless applications with fast startup times and low memory footprint.
Java EE (Enterprise Edition)
If you're working on larger, enterprise-level projects, Java EE offers a robust set of tools and APIs:
- JAX-RS (Java API for RESTful Web Services): Simplifies the creation of RESTful web services and clients.
- EJB (Enterprise JavaBeans): Provides a scalable, transactional, and multi-tiered architecture for building enterprise applications.
- JSF (JavaServer Faces): A framework for building component-based user interfaces for web applications.
- JPA (Java Persistence API): Manages relational data in applications using object-relational mapping.
- CDI (Contexts and Dependency Injection): Helps manage the lifecycle and interactions of stateful components in a loosely coupled way.
Quarkus
A Kubernetes-native Java stack, Quarkus is well-suited for cloud-native applications. It stands out for its fast startup times and low memory consumption, making it an excellent choice for microservices. Some of the frameworks it includes are:
- SmallRye MicroProfile: Implements the MicroProfile specifications to optimize Java EE applications for microservices architectures.
- RESTEasy: A REST framework to build web services and RESTful applications.
- Hibernate ORM with Panache: Simplifies the development of data access layers with a more user-friendly API.
- Vert.x: Provides a toolkit for building reactive applications on the JVM.
- Kafka: Integrates with Apache Kafka to handle real-time data feeds and streaming applications.
Each of these frameworks has its strengths and is well-suited for specific use cases. In our case, we will use Spring Boot.
Setting Up Spring Boot
There are multiple ways to create a spring boot project. Here we use Spring Initializr.
1. Create a New Spring Boot Project with Spring Initializr:
- Go to the Spring Initializr.
- Configure your project:
- Project: Maven or Gradle
- Language: Java
- Spring Boot Version: Select the latest stable version
- Project Metadata:
- Group: com.example
- Artifact: my-spring-boot-app
- Name: my-spring-boot-app
- Packaging: Jar
- Java Version: 11 or later
2. Add Dependencies:
- In the Dependencies section, add:
- Spring Web
- Spring Data JPA
- H2 Database (for an in-memory database)
- Add additional dependencies according to your project's needs.
3. Generate the Project:
- Click the "Generate" button to download a ZIP file containing your new Spring Boot project.
- Extract the ZIP file to your desired location.
4. Import the Project into Your IDE
- Open Your IDE:
- Launch IntelliJ IDEA, Eclipse, or your preferred IDE.
- Import the Project:
- In IntelliJ IDEA:
- Click File > Open and select the project folder.
- In Eclipse:
- Click File > Import > Existing Maven Projects and select the project folder.
- In IntelliJ IDEA:
Understand the Project Structure
Once you have your Spring Boot project set up, it's essential to understand its structure. Here's a breakdown of the key components:
Explanation:
- The @RestController annotation indicates that this class will handle HTTP requests and return JSON responses.
- The @GetMapping("/hello") annotation maps HTTP GET requests to the /hello URL path to the hello() method.
- The hello() method returns a simple "Hello World" message as a response.
Database Integration
To make your Spring Boot application more dynamic, you can integrate it with a database. In this section, we will set up a simple in-memory H2 database to store and retrieve the custom messages. This will demonstrate how to perform basic CRUD operations using Spring Data JPA.
By following these steps, we have integrated an H2 database into your Spring Boot application and set up basic CRUD operations to save and retrieve a custom message. This foundation can be expanded to include more complex data interactions and business logic.
Security and Authentication
Securing your Java backend is a top priority. You'll want to protect your APIs and sensitive data. Common security measures include:
Implementing authentication with tools like JSON Web Tokens (JWT).
Setting up authorization rules to control access to specific API endpoints.
Protecting against common web vulnerabilities like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF).
Security is a vast and crucial topic, so take the time to understand and implement it thoroughly.
In this section, we’ll create a simple security configuration using Spring Security and Basic Auth for authentication.
By completing these steps, we now have a secure and functional Java backend that can handle data storage and API requests. The next step is to integrate these APIs with our React frontend to create a complete full-stack application.
Developing the Reactjs Frontend with API Integration
When it comes to crafting your React frontend, incorporating general coding knowledge and UI principles is crucial. Bhargav Bachina, founder of Bachina Labs, recommends using React with a Java backend for dynamic web apps. Utilize Spring Security, deploy on AWS, and build React components to create a powerful user experience. Don't forget to consider user interface design and responsive CSS for an engaging frontend.
We'll now focus on integrating a React frontend with the Java backend we created. This is not a React learning tutorial, so we won't delve deeply into React concepts. Instead, we'll concentrate on how to connect your React app with the Java Spring Boot APIs we've built. We already covered how to set up a react project. Now, let's focus on how to connect our React frontend to the Java backend we created.
Communicating with the Backend
To fetch data from your Java backend, you'll make API requests from your React components. Axios, Fetch API, or libraries like Redux can help manage data fetching and state management.
Here's a simple example of making an API request in a React component using Axios:
User Interface and User Experience
Creating an intuitive and visually appealing user interface is essential for a successful web application. Consider the following aspects:
Choose a responsive design to ensure your app works well on various devices and screen sizes.
Utilize popular UI libraries like Material-UI or Ant Design to streamline your UI development.
Focus on user experience (UX) principles, such as fast load times, smooth transitions, and clear navigation.
A well-designed and user-friendly front end can set your application apart from the competition.
How CodeWalnut Could Help you in Integrating Java with React frontend
Integrating Java with React is a pivotal step in building modern web applications. CodeWalnut, a trusted name in software development, plays a vital role in bridging these technologies. With a team of experienced Java developers and React enthusiasts, CodeWalnut simplifies the fusion of these stacks, empowering your web projects. Whether you're crafting a Spring Boot app, running React UI components, or securing your application with Spring Security, CodeWalnut's expertise shines through. By seamlessly integrating Java's backend capabilities and React's dynamic front-end prowess, CodeWalnut ensures your web apps are efficient and responsive. Trust them to create user interfaces and databases using Spring Boot, elevating your web development game.
Key TakeAways
Seamless Integration: Combining Java with React offers a robust and dynamic solution for web application development.
Spring Boot Backend: Spring Boot is an excellent choice for building a Java backend due to its simplicity and efficiency.
User Interface: Develop an intuitive and responsive user interface in React to enhance the user experience.
API Communication: Use JavaScript to facilitate communication between your React frontend and Java backend, enabling data exchange.
FAQ
1: How to Build a Full-Stack React App with a Java Spring Boot Backend?
To build a full-stack React app with a Java Spring Boot backend, you'll need to leverage the power of these two technologies. Spring Boot provides a robust backend framework, and React.js offers a dynamic frontend. You can start by creating a Spring Boot application using Spring Initializr, setting up your RESTful APIs, and implementing CRUD operations. On the React side, use Create React App to create the frontend, and employ React Router for navigation. By integrating the backend and frontend, you can create a full-stack application that serves as a great foundation for more complex projects.
2: What Are the Key Steps in Combining React.js and Spring Boot for a Full-Stack Web App?
Combining React.js and Spring Boot to build a full-stack web app involves several key steps. First, set up your Spring Boot project using Spring Initializr, including the necessary dependencies such as Spring Data for data access and Spring Security for security features. Create RESTful APIs to serve as the backend for your app. Then, use Create React App to generate your React frontend and make API calls to interact with the Spring Boot backend. Employ React Router for client-side routing and create UI components to present your data. Secure your app with Spring Security and handle authentication and authorization. This synergy between React and Spring Boot enables you to build powerful full-stack applications.
3: Can You Explain the Role of Spring Security in a React and Spring Boot Application?
In a React and Spring Boot application, Spring Security plays a crucial role in ensuring the security of your web application. It provides features like authentication, authorization, and protection against common web vulnerabilities such as CSRF (Cross-Site Request Forgery). By integrating Spring Security into your application, you can manage user authentication, secure your RESTful endpoints, and control access to different parts of your app. This is essential for protecting sensitive data and maintaining the integrity of your full-stack application.
4: How to Add React Components to a Spring Boot Application?
Adding React components to a Spring Boot application involves creating reusable pieces of UI that can be embedded in your frontend. To do this, first, build your React components in the `src` directory of your React app. Once you have your components ready, you can add them to your Spring Boot application by including the necessary HTML tags in your Thymeleaf templates or by serving the React app as a static resource. Make sure to import the appropriate CSS files and JavaScript code in your Spring Boot application to fully utilize the React components.
5: How to Secure a Full-Stack Java and React App Using OAuth and Okta?
To secure your full-stack Java and React app using OAuth and Okta, you can follow a step-by-step tutorial on GitHub. Okta provides a convenient way to handle authentication and authorization in your application. You'll use Okta's OAuth services to manage user identity and access control. By configuring Okta in your app, you can implement single sign-on (SSO), ensuring that only authenticated users can access your resources. This enhances the security of your app and simplifies user management.
6: What Are the Best Practices for Building a Simple CRUD Application with Spring Boot and React?
Building a simple CRUD application with Spring Boot and React involves following best practices. Start by creating a Spring Boot application with Spring Data, which simplifies database operations. Define your entity classes and repository interfaces to interact with the database. On the React side, use React Router for navigation and build components for Create, Read, Update, and Delete operations. Ensure proper error handling and validation. Maintain clean code separation between your backend and frontend for a scalable and maintainable application.
7: How Can I Handle the Business Logic in a Full-Stack Java and React Application?
Handling business logic in a full-stack Java and React application typically involves placing the core logic in the Spring Boot backend. Your React frontend should primarily focus on user interface components and client-side interactions. Use Spring MVC controllers in the backend to receive requests from the frontend, process data, and return results. By centralizing the business logic in the backend, you can ensure data consistency and maintain a clear separation of concerns between frontend and backend, making your application more organized and easier to maintain.
8: What Are the Steps to Integrate Bootstrap's CSS and Reactstrap's Components with a React and Spring Boot Project?
To integrate Bootstrap's CSS and Reactstrap's components with your React and Spring Boot project, first import the necessary stylesheets and scripts into your React application. You can do this by including the links to Bootstrap's CSS and JavaScript files in your HTML templates. Next, install Reactstrap and add its components to your React components. Reactstrap provides pre-built components styled with Bootstrap, which you can customize as needed. This integration allows you to create a visually appealing user interface for your full-stack application while leveraging the power of React and Spring Boot for the backend functionality.
Get in touch - Our team has developed scalable solutions for enterprises and has a Crunch rating of 4.9⭐.
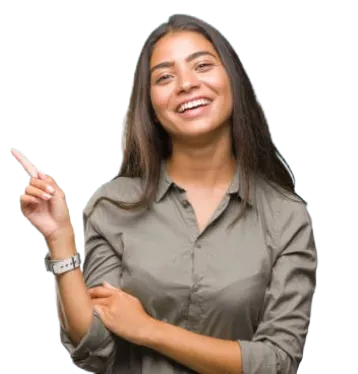
Experience coding prowess firsthand. Choose CodeWalnut to build a prototype within a week and make your choice with confidence.
Accelerate your web app vision with CodeWalnut. In just a week, we'll shape your idea into a polished prototype, powered by Vercel. Ready to make it real? Choose us with confidence!
Dreaming of a powerful web app on Heroku? Let CodeWalnut bring it to life in just one week. Take the leap and trust us to deliver with confidence!