How to Write Automation Test Cases in Selenium
Selenium is a versatile framework, supporting multiple programming languages like Java, Python, and C#. This versatility makes it an excellent choice for automation testing because it accommodates the preferences and expertise of various teams and individuals.
In today's fast-paced world of software development, the need for efficient and reliable testing methods has never been greater. This is where automation testing comes into play, and one of the leading tools in this domain is Selenium. In this comprehensive guide, we'll walk you through the process of writing automation test cases in Selenium, from the basics of Selenium to advanced testing techniques. By the end of this article, you'll be well-equipped to harness the power of Selenium and streamline your testing processes.
Quick Takeaways:
- Selenium is a robust open-source testing framework supporting multiple programming languages and offering automation for web applications.
- Selenium 4 brings enhancements like native support for multiple browser tabs, improved WebDriver API integration, and enhanced debugging features.
- Selenium offers benefits such as increased efficiency, code reusability, accuracy, parallel testing, cost savings, and seamless CI/CD integration, making it a valuable tool for high-quality web application testing.
Introduction to Selenium

Before diving into the world of automation test cases, it's crucial to acquaint ourselves with Selenium. Selenium is not just any testing framework; it's an open-source powerhouse that equips testers and developers with an array of tools for automating web applications. Its charm lies in its ability to interact with web elements, execute actions, and verify outcomes, all from within a web browser.
Selenium is a versatile framework, supporting multiple programming languages like Java, Python, and C#. This versatility makes it an excellent choice for automation testing because it accommodates the preferences and expertise of various teams and individuals.
What's New in Selenium 4?
The world of technology never stands still, and Selenium is no exception. The latest iteration, Selenium 4, brings with it a host of enhancements and features that make it an even more attractive option for automation testers.
One standout improvement in Selenium 4 is its native support for automating web applications in multiple browser tabs. This feature is a game-changer for testers working with modern web applications that rely heavily on tabbed interfaces.
Selenium 4 also boasts better integration with the WebDriver API, providing testers with more control and flexibility when automating tests. Additionally, debugging capabilities have been significantly enhanced, making it easier to identify and rectify issues in your test scripts.
These new features and enhancements cement Selenium 4's position as a valuable asset for automation testers seeking to streamline their testing processes.
- According to a 2023 survey by TestRail, Selenium is the most popular test automation framework, with over 70% of testers using it.
- The same survey found that the top three benefits of using Selenium for automation testing are:
- Increased test coverage
- Reduced testing time
- Improved test quality
- Another survey by Sauce Labs found that the top three challenges of writing automation test cases in Selenium are:
- Maintaining test cases
- Locating elements
- Handling dynamic content
Here are some tips for writing effective Selenium test cases:
- Start by understanding the application under test. What are the main features and functionality? What are the different user flows?
- Identify the key areas to test. This could include the login page, the checkout process, and any critical features.
- Write clear and concise test cases. Each test case should focus on a single test objective.
- Use descriptive and meaningful variable names. This will make your test cases more readable and maintainable.
- Use locators that are unique and stable. This will help to avoid test failures due to elements not being found.
- Handle dynamic content and wait times appropriately.
- Add assertions to verify that the expected results are achieved.
- Review and test your test cases thoroughly.
Here are some additional tips for writing effective Selenium test cases:
- Use a test automation framework. A test automation framework will provide you with a structure for writing and organizing your test cases. It will also make it easier to maintain and execute your test cases.
- Use a data-driven approach. A data-driven approach allows you to reuse your test cases with different data sets. This can save you a lot of time and effort.
- Use parallel testing. Parallel testing allows you to execute your test cases on multiple browsers and devices simultaneously. This can significantly reduce the time it takes to run your tests.
- Integrate your test cases with your CI/CD pipeline. This will allow you to run your tests automatically every time you make a change to your code.
Benefits of Selenium for Automation Testing

Why choose Selenium for your automation testing needs? Let's explore the numerous benefits it brings to the table:
- Efficiency: Selenium's automation capabilities allow for the rapid execution of test cases, significantly reducing testing time and boosting overall efficiency.
- Reusability: Test scripts created with Selenium are highly reusable. You can employ the same scripts across various test scenarios, promoting code efficiency and reducing redundancy.
- Accuracy: Automation eliminates the possibility of human error in repetitive testing tasks. Your test scripts execute the same actions with precision every time.
- Parallel Testing: Selenium facilitates parallel testing across multiple browsers and platforms, allowing you to validate your web application's compatibility effortlessly.
- Cost Savings: By automating testing processes, you reduce the need for manual testing, resulting in substantial cost savings over time.
- Continuous Integration (CI): Selenium can be seamlessly integrated into CI/CD pipelines, ensuring that tests run automatically as part of the development and deployment process.
Now that we've recognized the significance of Selenium, it's time to delve into the practical aspects of writing automation test cases.
Getting Started with Selenium
Before you can start crafting automation test cases, you must understand the various types of tests you can perform with Selenium:
- Functional Tests: These tests verify that individual functions of the application work as expected. They focus on specific functionalities, ensuring they perform as intended.
- Regression Tests: Regression tests are essential to ensure that new code changes haven't adversely affected existing functionality. They help maintain the application's stability.
- Load Tests: Load tests assess how the application performs under varying levels of load or traffic. This is crucial for applications that need to handle high user volumes.
- Integration Tests: Integration tests examine the interactions between different components or modules of the application. They ensure that different parts of the system work together seamlessly.
- Unit Tests: Unit tests are focused on testing individual units or components of the application in isolation. They help identify and fix issues at a granular level.
Selecting the Right Programming Language
Selenium offers support for multiple programming languages, including Java, Python, and C#. The choice of programming language depends on several factors, including your team's expertise and project requirements.
For example, if your team is well-versed in Java, it makes sense to use Java in conjunction with Selenium. Similarly, Python is an excellent choice due to its simplicity and readability, making it a popular language for Selenium automation.
Prerequisites for Your First Selenium Test
Before you can dive into writing automation test cases, you need to ensure that your testing environment is properly set up. Here are the essential prerequisites:
- Selenium WebDriver: Download and configure the Selenium WebDriver according to your chosen programming language. The WebDriver acts as the bridge between your test scripts and the web browser.
- Integrated Development Environment (IDE): Install an integrated development environment such as Eclipse or Visual Studio Code. These IDEs provide a comfortable workspace for writing and managing your test scripts.
- Build Tool: Utilize a build tool like Maven or Gradle for managing dependencies and building your automation framework.
- Web Browser: Ensure that you have the web browsers you intend to test (e.g., Chrome, Firefox) installed and up to date.
Configuring and Writing Selenium Test Scripts
How to Write Selenium Test Scripts
Selenium is a powerful tool for automating web application testing, enabling testers to save time and ensure consistent test execution. To harness the full potential of Selenium, it's essential to create effective test scripts. In this guide, we'll walk you through the process of crafting Selenium test scripts step by step.
Step 1: Prepare Your Components
Before diving into Selenium test script creation, it's crucial to ensure that all the necessary components are ready. These components typically include your development environment, Selenium WebDriver, and the web browser you plan to automate tests on.
First and foremost, you should have a working development environment set up. Depending on your choice of programming language for Selenium (such as Java, Python, or C#), you'll need to install the appropriate development tools and IDEs (Integrated Development Environments). Additionally, make sure you have Java Development Kit (JDK) installed if you opt for Java.
Next, ensure you have the Selenium WebDriver set up correctly. The WebDriver acts as a bridge between your test scripts and the web browser, allowing you to automate interactions with web elements. WebDriver libraries are available for various programming languages and browsers.
Lastly, ensure that you have the web browser you intend to test with installed and updated to the latest version. Selenium supports a wide range of browsers, including Chrome, Firefox, Safari, Edge, and more.
Step 2: Create a BrowserStack Account
BrowserStack is a cloud-based platform that facilitates cross-browser testing and helps you run Selenium test scripts in parallel on multiple browsers and devices. To leverage the power of BrowserStack, you need to sign up for an account.
Visit the BrowserStack website and register for an account by providing your details. Once registered, you'll have access to the BrowserStack platform, which offers a user-friendly interface for configuring, managing, and executing your Selenium tests.
Step 3: Access the Automate Tab
Within the BrowserStack platform, navigate to the 'Automate' tab, which is a dedicated section for Selenium automation testing. The Automate tab provides a comprehensive environment for configuring and running Selenium tests across various browsers and devices.
Here, you can set up your test environments, define desired capabilities, and manage your test suites effectively. BrowserStack simplifies the process of running Selenium tests at scale, making it a valuable addition to your test automation toolkit.
Step 4: Incorporate Essential Code
With your development environment ready, Selenium WebDriver configured, and BrowserStack account set up, it's time to start writing the essential code for your test scripts. The actual coding part will depend on your specific testing requirements, web application, and the programming language you've chosen.
Your Selenium test scripts will typically consist of a series of commands and actions performed on web elements within the web application. Selenium provides a rich set of commands and methods for interacting with elements like buttons, forms, links, and more. You'll also use assertions to verify that your web application behaves as expected.
To create a Selenium test script, you'll need to:
- Instantiate the WebDriver for your chosen browser.
- Navigate to the web page you want to test.
- Interact with web elements by locating them using various methods (by ID, name, XPath, etc.).
- Perform actions such as clicking buttons, entering text, or selecting options.
- Include assertions to verify the expected outcomes.
- Handle any exceptions or errors that may arise during test execution.
Remember to organize your test scripts logically, using functions or classes if applicable, to ensure maintainability and reusability.
Step 5: Integrate with BrowserStack
One of the key advantages of using BrowserStack is its seamless integration with Selenium. To integrate your Selenium test scripts with BrowserStack, you'll need to configure the desired capabilities in your code.
Capabilities define the browser, browser version, operating system, and other settings for your test execution. By specifying these capabilities, you can run your Selenium tests on various combinations of browsers and platforms provided by BrowserStack's extensive cloud infrastructure.
Integrating your test scripts with BrowserStack enables you to perform cross-browser testing effortlessly. You can ensure your web application functions correctly across different browsers and devices, all from a single test script.
Top 5 Tips for Effective Test Script Writing
To write effective Selenium test scripts, consider the following tips:
- Start With a Clear Test Plan: Define your testing objectives and outline your test cases before writing any code.
- Use Descriptive and Meaningful Names: Choose meaningful names for variables, methods, and test cases to enhance readability.
- Use Modular and Reusable Code: Break down your code into reusable functions and modules to minimize redundancy.
- Include Assertions in Your Tests: Implement assertions to validate that your web application functions correctly.
- Use Debugging and Logging: Debugging tools and logging can help troubleshoot issues and track the test execution process.
Troubleshooting Selenium Test Cases
Despite careful script writing, issues may arise during test execution. Here are some best practices for troubleshooting Selenium test cases:
- Inspect Elements: Verify that locators for web elements are correct.
- Check Waits: Ensure appropriate waits are in place for element visibility and interactions.
- Review Logs: Examine test logs for error messages and exceptions.
- Browser Compatibility: Confirm that tests run consistently across different browsers.
- Update WebDriver: Keep WebDriver and browser versions up to date.
Integrating Selenium with Other Testing Tools
Selenium can be integrated with other testing tools to enhance test coverage and effectiveness. Some popular integrations include:
- TestNG: A testing framework for parallel test execution and reporting.
- JUnit: A popular testing framework for Java applications.
- Cucumber: A behavior-driven development tool for creating test cases in natural language.
- Extent Reports: A reporting library for generating interactive test reports.
Advanced Selenium Testing Techniques
As you gain proficiency with Selenium, consider exploring advanced testing techniques, including:
- Visual Testing: Automate visual comparisons to identify UI differences.
- Parallel Testing: Execute multiple test cases simultaneously for faster results.
- Data-Driven Testing: Use external data sources to drive test scenarios.
- Page Object Model (POM): Organize your code into reusable Page Objects for better maintainability.
- Continuous Integration: Integrate Selenium tests into your CI/CD pipeline for automated testing.
In conclusion, mastering the art of crafting automation test cases in Selenium is more than just a skill—it's a key asset for ensuring the impeccable quality and unwavering reliability of web applications. With the right approach, a well-defined test plan, and the creation of effective test scripts using Selenium, your team can harness the full potential of this open-source powerhouse.
Selenium's versatility, supporting various programming languages like Java, enables you to tailor your testing framework to your specific project needs. Whether you're creating your first Selenium test script or configuring Selenium WebDriver for automated testing, you have a robust set of tools at your disposal.
As you embark on your journey to automate testing processes, remember that Selenium empowers you to eliminate human error, accelerate testing cycles, and deliver high-quality software with precision. It enables you to run tests across multiple browsers, ensuring your web application performs consistently, and it integrates seamlessly into your CI/CD pipelines, enhancing your development workflow.
Furthermore, if you're seeking expert guidance and resources to streamline your Selenium learning and implementation process, consider the support offered by Code Walnut. Our platform is designed to assist learners of all backgrounds, from seasoned developers looking to enhance their skills to newcomers to web development. We provide comprehensive resources, expertly designed courses, and hands-on projects, ensuring you have the knowledge and tools you need to excel in Selenium automation testing.
So, as you dive into writing your first test cases using Selenium, remember that with the right resources and support, your journey will be more efficient and rewarding. Let Selenium be your guiding light in the world of software testing, and let Code Walnut be your trusted ally in achieving testing excellence. Happy testing!
Get in touch - Our team has developed scalable solutions for enterprises and has a Crunch rating of 4.9⭐.
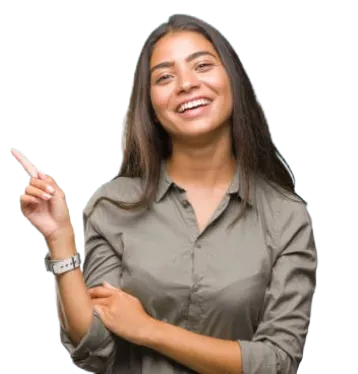
Experience coding prowess firsthand. Choose CodeWalnut to build a prototype within a week and make your choice with confidence.
Accelerate your web app vision with CodeWalnut. In just a week, we'll shape your idea into a polished prototype, powered by Vercel. Ready to make it real? Choose us with confidence!
Dreaming of a powerful web app on Heroku? Let CodeWalnut bring it to life in just one week. Take the leap and trust us to deliver with confidence!