Hey there, Welcome to one of the informative blog post. Today, we're diving deep into the world of ReactJS, and we've got an exciting topic: Higher-Order Components (HOCs). If you're a React developer or just someone curious about web development, you're in the right place.
In this article, we'll unravel the mysteries of Higher-Order Components, exploring what they are, how they differ from regular components, and the immense benefits they bring to your React applications. By the end of this read, you'll not only understand HOCs but also know how to wield them effectively in your projects.
So, without further ado, let's get started on this enlightening journey into the realm of Higher-Order Components.
What is Higher-Order Component in ReactJS?

To comprehend Higher-Order Components (HOCs), let's break it down into simpler terms. In ReactJS, components are the building blocks of your user interface. They encapsulate a piece of the UI, containing the logic and rendering information. You can think of them as reusable, self-contained code modules.
Now, a Higher-Order Component is like a power-up for these components. It's not a component itself, but rather a function that takes a component and returns a new enhanced component. Think of it as giving your regular component a boost, supercharging it with extra capabilities.
In essence, HOCs allow you to abstract common functionalities from components and reuse them across different parts of your application. This results in cleaner, more maintainable code, and it follows the DRY (Don't Repeat Yourself) principle, a fundamental concept in software development.
Difference between Component Development with and without Higher-Order Component in ReactJS?
To truly grasp the significance of Higher-Order Components, let's compare component development with and without them.
Component Development Without HOCs
When you develop React components without HOCs, you often find yourself duplicating code. Imagine you have several components that all need to access some user authentication data from your server. Without HOCs, you might end up repeating the same API calls and data handling logic in each of these components. This leads to code bloat, increased maintenance efforts, and a higher chance of introducing bugs.
Component Development with HOCs
Now, let's switch gears and see how HOCs come to the rescue. With Higher-Order Components, you can encapsulate the authentication logic in a single HOC. This HOC can then be applied to any component that requires authentication. It's like having a magic wand that grants your components superpowers, allowing them to access the required data effortlessly.
In summary, the key difference is that HOCs promote code reusability and maintainability, reducing redundancy and enhancing the overall quality of your codebase.
6 Benefits of Using Higher-Order Components in React
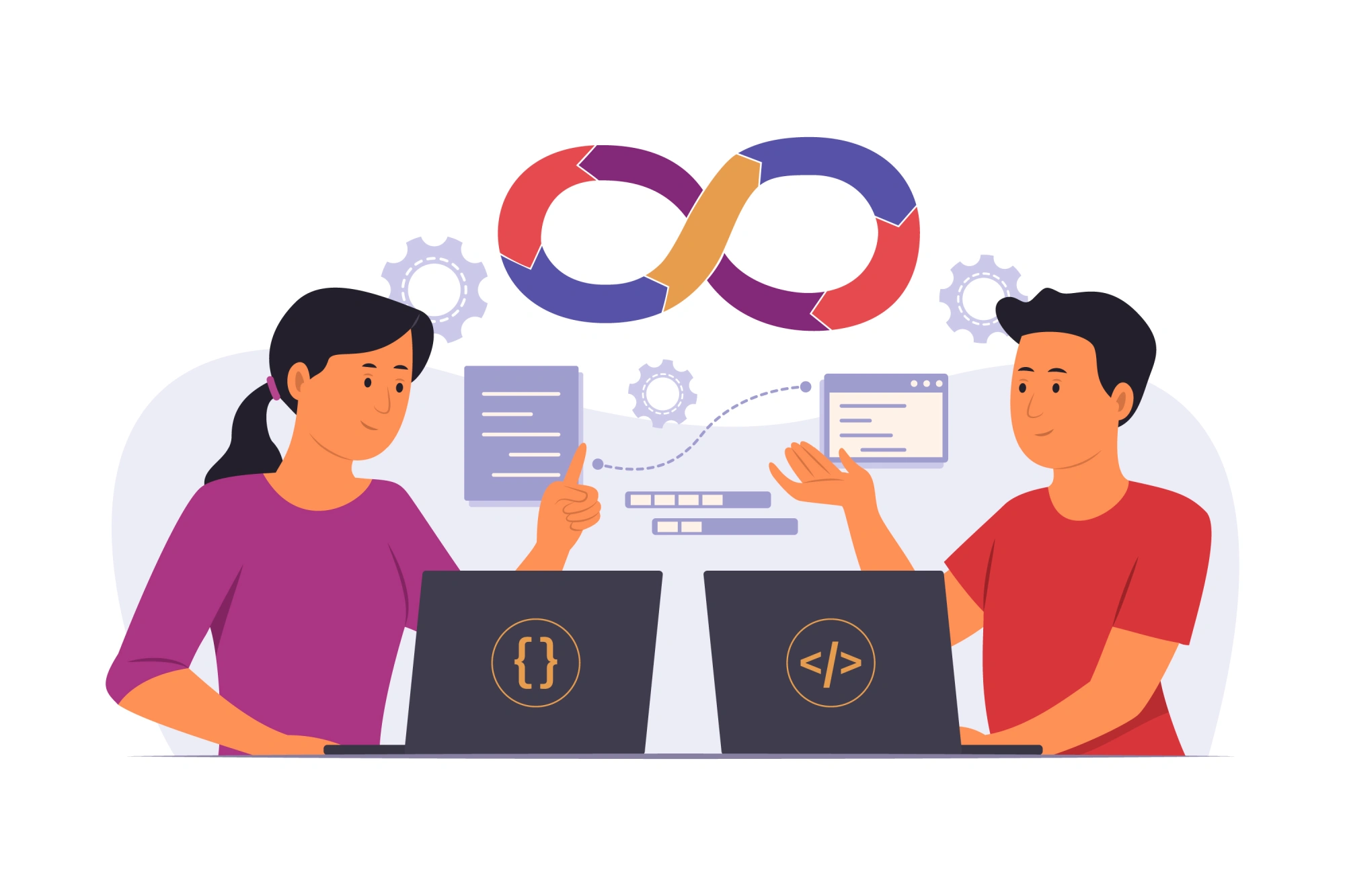
Now that we've established what HOCs are and how they differ from regular components, let's delve into the compelling benefits they offer.
1. Code Reusability
One of the primary advantages of Higher-Order Components is code reusability. By abstracting common functionalities into HOCs, you can use them across various components in your application. This not only saves time but also ensures consistency in your code.
2. Separation of Concerns
HOCs allow you to separate concerns within your application. For example, you can have an HOC responsible for handling data fetching and another for managing authentication. This separation makes your codebase more organized and easier to maintain.
3. Cleaner Component Code
When you use HOCs, your component code becomes cleaner and more focused. Components can focus on rendering and user interactions, while the complex logic can be handled by the HOCs. This leads to better component readability.
4. Improved Testing
HOCs can also enhance the testability of your components. Since you can isolate and test the HOCs independently, it becomes easier to write unit tests for different parts of your application.
5. Enhanced Scalability
As your application grows, HOCs become invaluable. They make it simpler to add new features or modify existing ones without extensively refactoring your components. This scalability is crucial for long-term project success.
6. Third-Party Integration
HOCs can be used to integrate third-party libraries or services into your components seamlessly. This is especially useful when working with APIs, state management, or other external resources.
With these benefits in mind, it's clear that Higher-Order Components can significantly improve your React development workflow and lead to more efficient, maintainable, and robust applications.
How to Use Higher-Order Components in ReactJS?
Now that you're eager to harness the power of HOCs, let's explore how to use them effectively in your React applications. We'll walk through the process step by step.
1. Create a Higher-Order Component
To begin, you'll need to create a Higher-Order Component. This is a function that takes a base component as an argument and returns an enhanced version of it. Here's a simplified example:
In this example, `withAuthentication` is the HOC. It takes `WrappedComponent` as its argument, which is the component you want to enhance with authentication.
2. Enhance Your Component
Next, you'll want to apply the HOC to the component you want to enhance. You do this by wrapping your component with the HOC, like so:
const EnhancedComponent = withAuthentication(BaseComponent);
Now, `EnhancedComponent` is the enhanced version of `BaseComponent` with authentication capabilities.
3. Use the Enhanced Component
Finally, you can use `EnhancedComponent` in your application just like any other component. It will inherit the functionalities provided by the HOC.
ReactDOM.render(<EnhancedComponent />, document.getElementById('root'));
And that's it! You've successfully incorporated a Higher-Order Component into your React application.
5 Higher-Order Component Conventions
Before we wrap up our journey into the world of Higher-Order Components, let's touch on some conventions and best practices to keep in mind when working with HOCs.
1. Naming
Choose descriptive and meaningful names for your HOCs. This makes it easier for other developers (or future you) to understand their purpose.
2. Pass Through Props
When enhancing a component with an HOC, make sure to pass through all props to the wrapped component. This ensures that the component behaves as expected and doesn't lose any functionality.
return <WrappedComponent {...this.props} />;
3. Avoid Mutating the Original Component
Never modify the original component inside an HOC. Always create a new component and return it, preserving the immutability of your components.
4. Keep HOCs Simple
HOCs are most effective when they have a single, well-defined responsibility. Avoid creating complex HOCs that try to do too much.
5. Document
Your HOCs, Just like any other part of your codebase, document your HOCs with comments or using documentation tools. Clear documentation makes it easier for others to understand and use your HOCs.
Key Takeaways
Congratulations, you've made it to the end of our journey through Higher-Order Components in ReactJS! Let's quickly recap what we've learned:
- Higher-Order Components (HOCs) are functions that enhance regular React components.
- They promote code reusability, separate concerns, and lead to cleaner, more maintainable code.
- You can create HOCs by wrapping a base component and returning an enhanced version of it.
- Follow naming conventions, pass through props, and keep HOCs simple for best practices.
And now, for a touch of humor: Remember, using HOCs in your React applications is like having a trusty sidekick. They may not wear capes, but they'll definitely save the day by reducing code redundancy and helping you build better, more scalable applications.
So, go ahead, level up your React game with Higher-Order Components, and watch your coding adventures unfold with greater ease and efficiency. Happy coding!
In the words of a wise developer (I won't force a quote, but you get the idea), "HOCs are your secret weapon in the world of React."
Coding for Success: How CodeWalnut Scaled Up with ReactJS Higher-Order Components
CodeWalnut, a forward-thinking company, has harnessed the power of Higher-Order Components (HOCs) in ReactJS to supercharge its web development. By employing HOCs effectively, CodeWalnut has streamlined its code, improved maintainability, and scaled its business operations. Discover how leveraging HOCs can elevate your React applications and drive business growth.
FAQ
What is a higher-order component (HOC)?
A higher-order component (HOC) is a function in React that takes a component as its argument and returns a new, enhanced component. This powerful concept allows you to add additional functionalities to your components without modifying their original code. HOCs are a fundamental part of modern React development and are commonly used to improve code reuse and maintainability.
How does a higher-order component work in React?
A higher-order component works by taking an existing component and wrapping it with another component. This wrapping component can provide additional props, behaviors, or logic to the original component. It essentially augments the capabilities of the original component.
Why are higher-order components in React important?
Higher-order components play a crucial role in enhancing the compositional nature of React. They allow you to create reusable logic and functionalities that can be applied to multiple components, promoting code reusability and making your codebase easier to maintain.
What are the primary applications of higher-order components in React?
Code Reusability: HOCs enable you to extract common functionalities and reuse them across various components, reducing redundancy in your code.
Separation of Concerns: HOCs help you separate different concerns within your application, such as data fetching, authentication, or UI rendering, into distinct components.
Improved Components: By using HOCs, your components become cleaner and more focused on their specific tasks, which enhances their readability and maintainability.
How does a higher-order component differ from a regular component in React?
A regular component in React is a self-contained unit responsible for rendering UI and managing its own state. In contrast, a higher-order component is a function that operates on one or more components, adding extra functionalities or modifying their behavior. HOCs are more like component enhancers.
How do you create a higher-order component in React?
To create an HOC, define a function that takes a component as its argument and returns a new component. Inside the HOC function, you can add logic, manipulate props, or perform other tasks that enhance the wrapped component's behavior.
How do you use higher-order components in a React project?
Using an HOC is as simple as wrapping your component with it. Here's an example of how to apply the `withAuthentication` HOC to an `App` component:
const EnhancedApp = withAuthentication(App);
Now, `EnhancedApp` is the enhanced version of the `App` component with authentication capabilities.
What are some best practices when working with higher-order components?
Naming: Choose descriptive names for your HOCs to make their purpose clear.
Passing Through Props: Ensure that all props are passed through from the HOC to the wrapped component to maintain expected functionality.
Avoid Mutations: Never modify the original component inside an HOC. Always return a new component to maintain immutability.
Documentation: Document your HOCs with comments or documentation tools to assist other developers in understanding their usage.
Can you give an example of using a higher-order component in React?
Certainly! Here's a simplified example of an authentication HOC:
Usage:
In this example, `withAuthentication` is the HOC that adds authentication logic to the `BaseComponent`, resulting in `EnhancedComponent`.
Are higher-order components the only way to achieve code reusability in React?
While higher-order components are a powerful way to achieve code reusability, React offers other techniques such as render props and hooks. The choice of which method to use depends on the specific requirements of your application and your coding preferences.
What are some advanced techniques for using higher-order components effectively?
Advanced techniques include creating container components with HOCs, composing multiple HOCs for complex functionalities, and using HOCs in combination with React hooks to manage state and side effects.
How do higher-order components relate to React's compositional nature?
Higher-order components align with React's compositional nature by allowing you to compose additional behaviors or features into components. This follows the principle of building larger components from smaller, reusable building blocks, which is a fundamental concept in React development.
Experience coding prowess firsthand. Choose CodeWalnut to build a prototype within a week and make your choice with confidence.
Accelerate your web app vision with CodeWalnut. In just a week, we'll shape your idea into a polished prototype, powered by Vercel. Ready to make it real? Choose us with confidence!
Dreaming of a powerful web app on Heroku? Let CodeWalnut bring it to life in just one week. Take the leap and trust us to deliver with confidence!