Hey there, fellow developers! 👋
Welcome back to another exciting journey through the world of web development. Today, we're going to dive deep into the realm of Next.js and explore a powerful tool known as SWR. If you've ever wondered how to supercharge your data fetching in Next.js and enhance your web applications' performance, you're in the right place.
In this comprehensive guide, we'll walk you through what SWR is, its advantages, and provide you with a step-by-step guide on how to use it effectively in your Next.js projects. By the end of this post, you'll be equipped with the knowledge and skills to take your Next.js development to the next level.
SWR Unveiled: Simplifying Data Fetching in Next.js
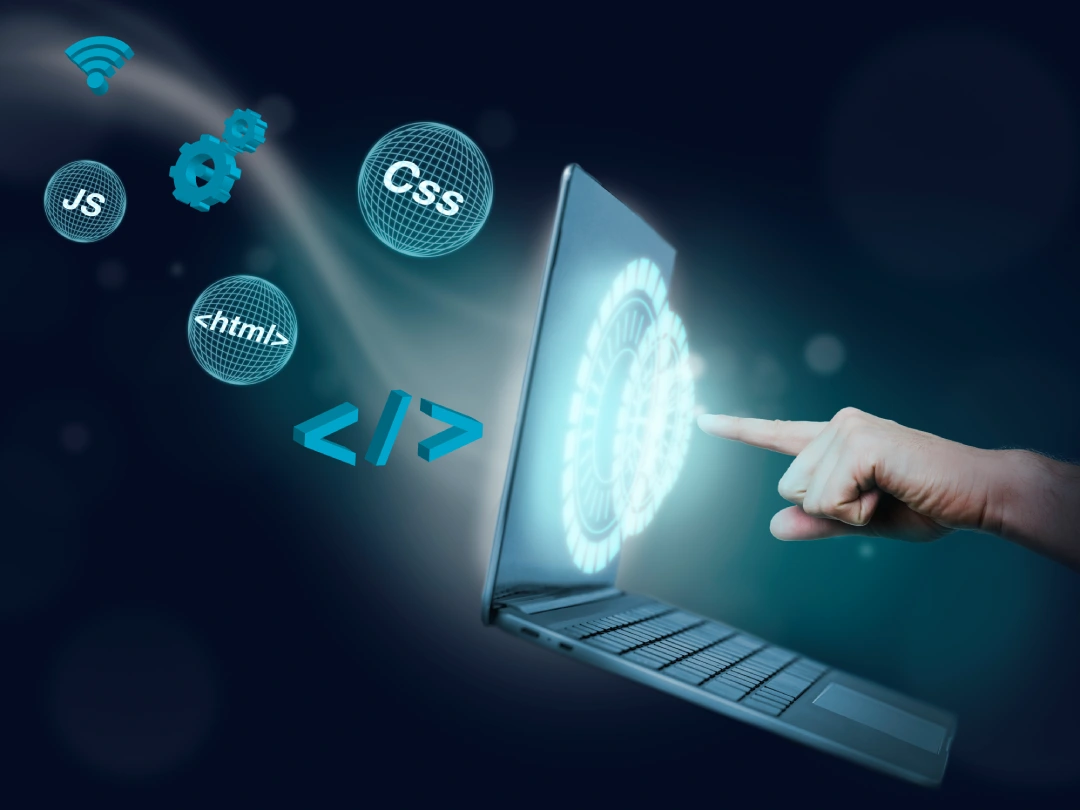
Let's start at the beginning—what exactly is SWR?
SWR stands for Stale-While-Revalidate and it's a data-fetching library for Next.js that simplifies the process of fetching and caching data from various sources. It's like a turbocharger for your Next.js applications, ensuring your data remains up-to-date while providing a smooth and responsive user experience.
Unlocking Next.js Potential: 4 Advantages of SWR
1. Effortless Data Fetching
One of the most significant advantages of using SWR is how effortlessly it enables data fetching. With just a few lines of code, you can fetch data from various sources like APIs, databases, or even your server, without breaking a sweat.
2. Automatic Caching
SWR intelligently caches your data. It means that once you've fetched data, it's readily available, reducing the need for redundant requests. This not only speeds up your application but also reduces the load on your server.
3. Real-time Updates
In a world where real-time data is crucial, SWR shines. It allows your application to automatically revalidate data in the background, ensuring your users always have access to the latest information.
4. Error Resilience
SWR is equipped to handle data fetching issues gracefully. It provides a way to deal with errors, ensuring a seamless user experience even when things don't go as planned.
Mastering SWR: A Step-by-Step Guide for Next.js
Now, let's roll up our sleeves and dive into the practical aspect of using SWR in Next.js. We'll walk you through the steps, starting with installation and moving on to more advanced features.
Installation
To get started with SWR in your Next.js project, follow these simple steps:
1. Install SWR via npm or yarn:
2. Import SWR in your Next.js component:
import useSWR from 'swr';
3. Fetch data using SWR:
const { data, error } = useSWR('/api/data', fetcher);
Here, `'/api/data'` is the endpoint you want to fetch data from, and `fetcher` is a function that handles the actual data retrieval.
Basic Usage
Let's take a closer look at a basic example of using SWR to fetch and display data in your Next.js component:
Here, we're fetching data from `/api/data` using the `fetcher` function and handling different states like loading and errors.
Supercharged Performance: Caching Strategies with SWR
SWR's caching strategies are where the magic happens. By default, SWR uses a "stale-while-revalidate" strategy, but you can customize it according to your specific needs.
Customizing Caching Strategies
You can set caching strategies with the `revalidateOnFocus`, `revalidateOnReconnect`, and `revalidateOnMount` options. For example, to revalidate data only when the user explicitly requests it, you can do:
This allows you to fine-tune your application's performance based on your use case.
Real-Time Data Updates: Revalidation with SWR
In a rapidly changing world, keeping your data up-to-date is essential. SWR offers automatic revalidation, ensuring your data remains fresh without the need for manual refreshes.
By setting the `refreshInterval` option, you can specify how often SWR should check for updates and revalidate your data.
Responsive and Dynamic: Optimistic UI Updates with SWR
Optimistic UI updates make your application feel more responsive by updating the user interface optimistically before the server confirms the change. SWR can help you implement this functionality seamlessly.
In this example, we're optimistically updating the UI with a new post before confirming the creation with the server.
Error Resilience: Handling Data Fetching Issues with SWR
No application is without its hiccups. SWR provides a robust way to handle data fetching errors gracefully.
By checking the `error` state, you can provide helpful feedback to users when something goes wrong.
Seamless Integration: Using SWR with Next.js APIs
Integrating SWR with Next.js APIs is a match made in heaven. You can easily create powerful serverless APIs and fetch data with SWR.
Here's a quick example of how to create a serverless API endpoint in Next.js and fetch data from it using SWR:
Now, you can use SWR to fetch data from this API endpoint just like you would with any other API.
Third-Party Data Magic: Harnessing SWR with External APIs
SWR isn't limited to fetching data from your own server. You can use it to retrieve information from external APIs as well. Let's say you want to fetch weather data from a third-party API:
Here, we're fetching weather data from a third-party API and displaying it in our Next.js application.
SWR Success: Best Practices for Next-Level Next.js Development
As we wrap up our journey through SWR in Next.js, let's take a moment to recap some best practices:
Keep It DRY (Don't Repeat Yourself): Reuse SWR hooks and components to avoid duplicating code.
Fine-Tune Caching Strategies: Customize caching strategies to optimize performance for your specific use case.
Optimistic UI Updates: Implement optimistic UI updates for a smoother user experience.
Error Handling: Gracefully handle data fetching errors with clear user feedback.
External APIs: Utilize SWR to fetch data from external APIs seamlessly.
Stay Updated: Keep an eye on the Next.js and SWR documentation for updates and improvements.
Remember, mastering SWR takes practice, but once you do, it can significantly enhance your Next.js projects.
And there you have it, folks! We've journeyed through the world of SWR in Next.js, discovering its potential, advantages, and how to use it effectively. Now, it's your turn to unleash the power of SWR in your Next.js applications. Happy coding! 🚀
Key Takeaways
- SWR (Stale-While-Revalidate) simplifies data fetching in Next.js, providing automatic caching, real-time updates, and error resilience.
- Installation and basic usage of SWR in Next.js involve importing the library, fetching data, and handling different states.
- Customize caching strategies, implement optimistic UI updates, and gracefully handle errors for a seamless user experience.
- SWR can be integrated seamlessly with Next.js APIs and external APIs, expanding its use cases.
- Follow best practices to master SWR and supercharge your Next.js development.
And, as always, remember: When in doubt, SWR it out! 😉
CodeWalnut: Masters of SWR in Next.js for Data Fetching
At CodeWalnut, we've honed our skills in Next.js to perfection. Our team's expertise in SWR ensures super-efficient data fetching, resulting in lightning-fast and reliable web applications. Trust CodeWalnut for top-tier performance in your Next.js projects.
What is SWR in Next.js: FAQ
1. What is SWR in Next.js?
SWR stands for Stale-While-Revalidate, and it's a powerful library for data fetching in Next.js. It's designed to make client-side data fetching a breeze. SWR automatically handles data caching, ensuring your data remains up-to-date without unnecessary server requests.
2. How does SWR work in Next.js?
SWR is a React hook (you'll often see it referred to as `useSWR`) that simplifies data fetching. It fetches data using a provided fetcher function and caches the results. When you request data with SWR, it first returns cached data (if available), then sends a fetch request to get the latest data. This combination of cached and fresh data provides a smoother user experience.
3. When should I use SWR in my Next.js application?
You should consider using SWR in your Next.js project when:
- You want to fetch data on the client-side without the need for pre-rendering.
- Your application requires real-time data updates.
- You're working with large amounts of data, and you want to optimize performance.
- Data retrieval is dependent on other data, and you need a way to manage these dependencies.
- You're looking for a reusable data-fetching solution that works seamlessly with Next.js.
4. Can I use SWR with external APIs in Next.js?
Absolutely! SWR isn't limited to fetching data from your own server. You can use it to fetch data from external APIs as well. It's a versatile tool that helps you integrate and manage data from various sources, whether it's your server or third-party services.
5. How do I handle data fetching errors with SWR in Next.js?
SWR makes it easy to handle data fetching errors gracefully. When an error occurs while fetching data, SWR provides an error object that you can use to display an error message to the user. This ensures a smooth user experience even when things don't go as planned.
6. What are some best practices for using SWR in Next.js?
Here are some best practices when using SWR in Next.js:
- Fine-Tune Caching Strategies: Customize caching strategies to optimize performance for your specific use case.
- Optimistic UI Updates: Implement optimistic UI updates for a smoother user experience.
- Error Handling: Gracefully handle data fetching errors with clear user feedback.
- External APIs: Utilize SWR to fetch data from external APIs seamlessly.
- Stay Updated: Keep an eye on the Next.js and SWR documentation for updates and improvements.
By following these best practices, you can master SWR and supercharge your Next.js development.
7. Is SWR suitable for real-time data updates in Next.js?
Yes, SWR is excellent for real-time data updates in Next.js. It offers an automatic revalidation feature, which allows your application to automatically revalidate data in the background, ensuring your users always have access to the latest data.
8. How does SWR handle data that depends on other data in Next.js?
SWR provides a powerful way to manage data dependencies. You can use SWR hooks within SWR hooks to fetch data that depends on other data. SWR intelligently revalidates all related data, ensuring it's always up-to-date.
9. What's the key advantage of using SWR in Next.js?
The key advantage of using SWR in Next.js is its ability to simplify and streamline client-side data fetching. It provides a robust solution for caching, real-time updates, and error resilience, making your Next.js application more efficient and responsive.
10. How can I get started with SWR in Next.js?
To get started with SWR in Next.js, you'll need to install the library and import it into your components. You can then use the `useSWR` hook to fetch data from your desired source. Be sure to refer to the official documentation and examples for more detailed guidance.
That's a wrap for our SWR in Next.js FAQ! If you have more questions or need further assistance, feel free to explore the SWR documentation or reach out to the vibrant CodeWalnut community. Happy coding! 🚀
Experience coding prowess firsthand. Choose CodeWalnut to build a prototype within a week and make your choice with confidence.
Accelerate your web app vision with CodeWalnut. In just a week, we'll shape your idea into a polished prototype, powered by Vercel. Ready to make it real? Choose us with confidence!
Dreaming of a powerful web app on Heroku? Let CodeWalnut bring it to life in just one week. Take the leap and trust us to deliver with confidence!