How ReactJS Handles Immutability to Improve Performance
ReactJS plays a crucial role in crafting dynamic and interactive web experiences. This section explores how React achieves this by efficiently managing the state of user interfaces and responding to data changes.
In the ever-evolving world of web development, the need for high-performing web applications has become paramount. Users expect websites to load quickly and respond seamlessly to their interactions. Achieving these performance goals often hinges on how effectively a front-end framework manages data and updates the user interface. ReactJS, a popular JavaScript library for building user interfaces, tackles this challenge head-on by harnessing the power of immutability.
Quick Takeaways
- ReactJS values web performance and employs immutability to enhance web app responsiveness.
- React's performance strategy centers on immutable data structures and the Virtual DOM, delivering faster web applications.
- While immutability offers predictability and performance gains, it may also raise memory usage and pose a learning curve, requiring careful implementation decisions.
The Significance of Web Performance
Web performance is not a luxury; it's a necessity. In an era where user attention spans are dwindling, a slow or unresponsive web application can quickly drive users away. This section explores why web performance matters and why developers must prioritize it.
Unveiling ReactJS: The Powerhouse of UI Development
ReactJS has emerged as a powerhouse in the realm of UI development. Its component-based architecture, virtual DOM, and efficient rendering mechanisms have made it the go-to choice for building dynamic and responsive web applications. In this section, we'll take a closer look at what makes ReactJS so powerful.
Boosting React Performance with Immutability
To unlock React's full potential, developers must understand how it leverages immutability to boost performance. This section serves as an introduction to the core concept of immutability in React and sets the stage for a deeper exploration.
React's Role in Crafting Dynamic Web Experiences
ReactJS plays a crucial role in crafting dynamic and interactive web experiences. This section explores how React achieves this by efficiently managing the state of user interfaces and responding to data changes.
Tackling Performance Challenges in Web Development
Web development presents numerous performance challenges, from rendering complex user interfaces to efficiently updating the Document Object Model (DOM). This section discusses these challenges and how React addresses them.
Immutability in JavaScript: Embracing Immutability in JavaScript

Immutability, in the context of JavaScript, refers to the concept of creating data structures that cannot be modified after they are created. In this section, we'll explore the significance of immutability in JavaScript programming.
JavaScript, by default, allows for mutable data structures. This means that variables can change their values over time. While mutability can be convenient, it can also introduce complexity and unexpected behavior into your code.
Immutability, on the other hand, promotes a more predictable and reliable programming style. When data is immutable, you can be confident that it won't change unexpectedly, leading to fewer bugs and easier debugging.
We'll also discuss how immutability can improve code readability and maintainability. Immutable data makes it clear when and where changes occur, making your code easier to reason about.
The Pitfalls of Mutable Operations in JavaScript
Mutable operations in JavaScript can lead to unexpected behavior and hard-to-debug issues. This section will delve into the disadvantages of mutable operations and why embracing immutability is often a better approach.
Common mutable operations in JavaScript, such as modifying objects or arrays in place, can result in subtle bugs that are difficult to track down. For instance, if multiple parts of your codebase have access to the same mutable data and modify it, it can lead to unintended side effects.
We'll discuss scenarios where mutable operations can cause problems, including issues related to reference sharing, asynchronous code, and debugging challenges.
By understanding the pitfalls of mutability, developers can make informed decisions about when and where to use immutable data structures, especially in the context of React and web development.
Decoding React's Virtual DOM
React's Virtual DOM is a critical part of its performance optimization strategy. This section will provide a comprehensive understanding of what the Virtual DOM is, how it works, and its role in minimizing costly DOM manipulations.
The Virtual DOM is a lightweight, in-memory representation of the actual DOM. When changes occur in a React component, React first updates the Virtual DOM, which is much faster than directly manipulating the real DOM. React then calculates the difference between the Virtual DOM and the real DOM (known as "diffing") and applies the minimal set of changes needed to update the actual page.
We'll explain how React uses this process to ensure that UI updates are as efficient as possible, reducing rendering time and enhancing overall performance.
Harnessing the Virtual DOM for Lightning-Fast Rendering
The synergy between immutability and React's Virtual DOM is crucial for achieving exceptional performance. In this section, we'll explore how React utilizes immutability to create a virtual representation of the DOM and optimize updates.
React's Virtual DOM relies on the immutability of data to determine what has changed since the last update. By working with immutable data, React can quickly identify differences between previous and current states, resulting in minimal updates to the DOM.
We'll dive into practical examples of how immutability enables React to work efficiently with the Virtual DOM. This understanding will shed light on why immutability is a fundamental aspect of React's performance gains.
React's Love Affair with Immutable Data Structures
React's preference for immutable data structures is at the core of its approach to state management. In this section, we'll explore why React favors immutability and how it benefits developers.
Immutability ensures that once data is created, it cannot be changed. This predictability simplifies state management in React components, as developers can rely on the fact that data won't mutate unexpectedly.
We'll discuss how React's embrace of immutable data structures aligns with its philosophy of making UI updates more predictable, maintainable, and efficient.
Unpacking the Benefits of Immutable Data in React
Immutability offers a multitude of advantages, and in this section, we'll dissect these benefits and illustrate how they translate into a better React development experience.
Predictability: Immutable data ensures that data remains consistent and doesn't change unexpectedly. This predictability simplifies debugging and reduces the likelihood of bugs related to state changes.
Easier Debugging: When an issue arises, it's easier to track down the source of the problem in code that relies on immutable data, as you can trust that data won't change unexpectedly.
Enhanced Performance: Immutable data enables React to optimize rendering by reducing unnecessary re-renders, resulting in better performance for your applications.
We'll provide concrete examples and scenarios to demonstrate how these benefits play out in practice, highlighting why immutability is a valuable asset in React development.
A Journey Through Immutable Data Handling in React
Practical examples are often the most effective way to understand complex concepts. In this section, we'll embark on a journey through various scenarios to illustrate how React handles immutable data, from state management to prop handling.
We'll explore real-world examples of how to work with immutable data in React components, emphasizing best practices and demonstrating how to leverage immutability effectively.
By the end of this section, readers will have a clear understanding of how to apply immutable data handling in their own React projects, enhancing the performance and maintainability of their applications.
These sections collectively provide a comprehensive understanding of the significance of immutability in JavaScript and how it plays a crucial role in React's approach to improving performance.
Challenges and Pitfalls
While immutability offers significant benefits, it may also present challenges. In this section, we address common obstacles and pitfalls that developers may encounter when embracing immutability in React.
A. Navigating the Immutability Terrain: Challenges Ahead
This subsection explores potential challenges in implementing immutability and offers insights into how to navigate them successfully.
B. Conquering Challenges: Best Practices and Solutions
Challenges are meant to be overcome. Here, we provide practical solutions and best practices for conquering the hurdles associated with immutability in React development.
Immutability Libraries
The React team advocates for the use of libraries like Immutable.js and immutability-helper to incorporate immutability into your projects. However, there are numerous libraries available, each offering similar functionality. These libraries generally fall into three main categories:
- Specialized Data Structures: Some libraries are designed to work seamlessly with specialized data structures tailored for immutability.
- Object Freezing: Another category of libraries achieves immutability by freezing objects, preventing them from being modified after creation.
- Helper Functions: Several libraries provide a set of helper functions that facilitate immutable operations on data.
The majority of these libraries are built around the concept of persistent data structures.
Persistent Data Structures
Persistent data structures are designed to create a new version of the data whenever any modification occurs, ensuring the immutability of existing data while retaining access to all previous versions.
In the case of partially persistent data structures, you can access all previous versions, but modifications are limited to the newest version. In contrast, fully persistent data structures allow both access to and modification of every version.
The efficiency of persistent data structures hinges on two fundamental concepts: trees and sharing.
Under the hood, these data structures function as a list or a map, but their implementation relies on a specialized type of tree known as a bitmapped vector tree. In this structure, only the leaf nodes store values, while the binary representation of keys forms the inner nodes of the tree.
Benefits of Immutability
Immutability, the concept of data that cannot be changed once it's created, offers a range of benefits in modern web development:
- Predictability: Immutable data structures ensure that the state of your application remains consistent and doesn't change unexpectedly. This predictability simplifies debugging and reduces the likelihood of hard-to-trace bugs caused by mutable data.
- Easier Debugging: When issues arise in your code, it's easier to pinpoint the source of the problem when you work with immutable data. Since you can trust that the data won't change after creation, you can focus on the code that caused the issue, rather than wondering if data mutations are at fault.
- Concurrency: Immutable data is inherently thread-safe. In multi-threaded or concurrent applications, immutability eliminates the need for complex locking mechanisms, reducing the risk of data corruption and race conditions.
- Functional Programming: Immutability is a fundamental concept in functional programming, which has gained popularity in modern web development. Available programming promotes the use of pure functions and avoids side effects, making code easier to reason about, test, and maintain.
- Optimized Rendering: In ReactJS and similar libraries/frameworks, immutability enables efficient rendering. By knowing that data won't change, these libraries can minimize DOM updates, leading to faster and more responsive user interfaces.
Disadvantages of Immutability
While immutability offers significant advantages, it's essential to acknowledge its disadvantages as well:
- Memory Consumption: Immutable data structures often require more memory because creating a new copy of data every time a change is made can be resource-intensive, especially for large datasets.
- Performance Overheads: In some scenarios, the performance overhead of creating new copies of data can outweigh the benefits of immutability. For real-time applications or situations where memory is constrained, immutability might not be the best choice.
- Learning Curve: Embracing immutability can be a paradigm shift for developers accustomed to mutable data. Learning new patterns and practices, such as avoiding in-place updates, may require time and effort.
- Complexity in Updates: Updating immutable data can be less intuitive than modifying mutable data in place. In some cases, creating entirely new data structures for minor updates may lead to complex code.
- Compatibility: Integrating immutable data into existing codebases, especially those reliant on mutable data, can be challenging. This transition may require refactoring and adjustments to accommodate immutability.
- Limited Language Support: While some programming languages have built-in support for immutability (e.g., Clojure or Haskell), others may lack robust libraries or language features, making it more cumbersome to work with immutable data.
What Lies Ahead: Emerging Trends in React and Immutability
The landscape of web development is ever-evolving. In this section, we discuss emerging trends in React and immutability, offering insights into the future of this dynamic ecosystem.
Wrapping up
In this comprehensive exploration of how ReactJS handles immutability to improve performance, we've uncovered the inner workings of React's optimization strategies. From its reliance on immutable data structures to the practical benefits and real-world impact, immutability plays a pivotal role in React's success. As you apply these principles in your own React projects, you'll not only harness the power of immutability but also contribute to a faster and more efficient web. Embrace immutability, and empower your React applications for peak performance.
Get in touch - Our team has developed scalable solutions for enterprises and has a Crunch rating of 4.9⭐.
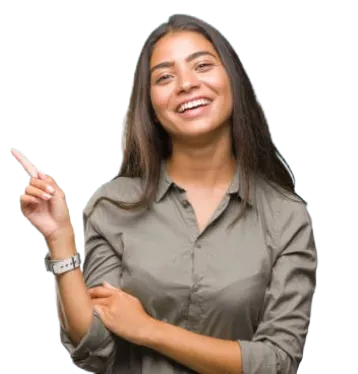
Experience coding prowess firsthand. Choose CodeWalnut to build a prototype within a week and make your choice with confidence.
Accelerate your web app vision with CodeWalnut. In just a week, we'll shape your idea into a polished prototype, powered by Vercel. Ready to make it real? Choose us with confidence!
Dreaming of a powerful web app on Heroku? Let CodeWalnut bring it to life in just one week. Take the leap and trust us to deliver with confidence!