React architecture best practices for 2023
A component-based architecture is the way to go, organizing your React project with a clear folder structure. Reuse components using naming conventions for better codebase organization. Utilize design patterns, higher-order components, and custom hooks to efficiently manage business logic. Implement CSS in JavaScript for maintainable styling.
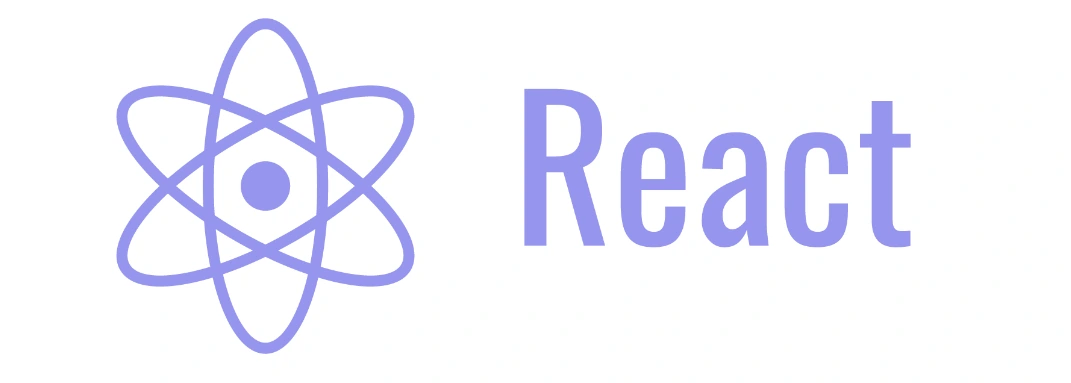
Hey there, fellow React enthusiasts!
Today, we're diving headfirst into the intricate world of React architecture. If you're aiming to craft efficient and scalable React applications, this is your one-stop guide.
In this in-depth journey, we'll cover the fundamental building blocks of React architecture and the best practices to create a solid foundation for your projects. I'm here to be your virtual tour guide, so let's get started.
Understanding the Fundamentals of React Architecture
What Is React and Why Does It Matter?
First things first, what exactly is React, and why should you care? React is a JavaScript library for building user interfaces. It's maintained by Facebook and a community of individual developers and companies. What sets React apart is its ability to create dynamic, interactive, and high-performance UIs with ease. Understanding React is crucial because it empowers you to create web applications that feel snappy and responsive. It’s like having the secret sauce to build amazing user experiences.
The Key Components of a React Application
Components in React serve as the DNA of your application, encapsulating specific functionality and UI elements. Functional components, favored for their simplicity and ease of testing, are ideal for most scenarios. On the other hand, class components, with their lifecycle methods, are better suited for complex state management and interactivity. The choice between them depends on your project's requirements and goals, and we'll guide you through making the right selection for a flexible and maintainable codebase.
The Importance of a Well-Defined Architecture
The importance of a well-defined architecture in any software development project cannot be overstated. A solid architectural foundation ensures that your project is organized, scalable, and maintainable. It enhances collaboration among team members, simplifies debugging, and accelerates development. A well-structured architecture is like a roadmap, guiding your project's growth and adaptability, ultimately leading to a more efficient and successful software product. Don't underestimate the power of a well-defined architecture – it's the key to long-term project success.
Choosing the Right React Component Structure
Organizing Components: Functional vs. Class Components
Container and Presentational Components: A Best Practice
In React architecture, the separation of container and presentational components is a best practice that can greatly enhance the maintainability and scalability of your applications. Container components, often referred to as smart components, handle data logic and state management. Presentational components, or dumb components, focus solely on the visual representation. This clear division of responsibilities not only simplifies code but also facilitates reusability and collaboration among developers. By adopting this practice, you can create more organized, efficient, and maintainable React applications.
The Role of Hooks in Modern React Architecture
React Hooks have fundamentally transformed the way we handle state and side effects in modern React applications. They offer a more elegant and functional approach to managing component logic compared to class components. With hooks, you can encapsulate state, effects, and context in a cleaner, more reusable manner. They provide an excellent solution for code organization and enable developers to create more readable and maintainable components. This article delves into the significance of hooks in shaping the architecture of modern React applications and their impact on development practices.
State Management Best Practices

Why Centralized State Management Matters
Centralized state management is crucial for maintaining clean, efficient code in React applications. By keeping data in a single, accessible repository, it ensures a predictable data flow, fosters consistency across components, and simplifies debugging and testing. As applications scale, this approach eases the complexity of state management. In summary, centralized state management is the linchpin of a well-organized, maintainable, and scalable React architecture.
Redux vs. Context API: Which One to Choose?
Immutability and Immutable Data Structures
Immutability, in the context of React and modern application development, is the practice of not changing data directly but instead creating new instances with updated values. Immutable data structures are fundamental to this concept. They ensure that data remains unchanged once it's created, reducing unexpected side effects and simplifying debugging. In React, immutability is critical for efficient state management and optimal rendering performance, particularly when working with large datasets. By understanding and applying immutable data structures, developers can create more reliable and predictable applications.
Effective Routing and Navigation
React Router: A Go-To Solution for Navigation
React Router is undeniably the go-to solution for handling navigation in your React applications. It offers a robust, declarative way to manage routing, ensuring your app's UI updates seamlessly as users navigate. With React Router, you can create dynamic, client-side navigation that feels like a native app. It simplifies route configuration, supports nested routes, and allows for flexible URL parameter handling. Whether you're building a single-page application or a complex web platform, React Router is your trusty companion for crafting seamless and user-friendly navigation experiences.
Handling Nested Routes and Layouts
Handling nested routes and layouts in React involves structuring your app's UI with a hierarchy of components. This method simplifies the management of complex layouts and views. Define route configurations, create layout components, and modularize your app. Consider implementing access control, optimize URL structure, manage state, and prepare for error handling. Thorough testing ensures seamless navigation and UI transitions, resulting in an organized, user-friendly, and maintainable application.
Performance Optimization Techniques
Code Splitting: Reducing Initial Load Times
Code splitting is a strategy that significantly improves your React app's initial load times by dividing the JavaScript bundle into smaller, more manageable pieces. By dynamically loading only the code needed for a specific page or feature, you reduce the initial load size, enhancing your app's performance. This technique can be implemented using tools like Webpack's dynamic imports or React's Suspense API. Code splitting is especially beneficial for large applications, ensuring that users experience faster load times and smoother interactions, ultimately leading to a better user experience.
Memoization and Performance Enhancements
Memoization is a performance-enhancing technique in React that optimizes rendering efficiency. By caching the results of costly function calls, it reduces redundant computations and minimizes re-rendering. React's `useMemo` hook, for instance, enables data caching and recalculation only when relevant dependencies change. This approach efficiently handles complex calculations, filtering, or sorting operations, resulting in a more responsive user interface. Memoization is a valuable tool for enhancing the performance of React applications, particularly when components manage data transformations, ensuring a smoother and more efficient user experience.
Key Takeaways
In 2023, following best practices for React architecture is essential. A component-based architecture is the way to go, organizing your React project with a clear folder structure. Reuse components using naming conventions for better codebase organization. Utilize design patterns, higher-order components, and custom hooks to efficiently manage business logic. Implement CSS in JavaScript for maintainable styling. For global state, React Context or libraries like Redux are recommended. As your React project grows, maintain a clear separation of logic from the UI, keeping your application structured and scalable.
How does CodeWalnut could help in implementing best React architecture
CodeWalnut plays a crucial role in developing React architecture based web for efficient and scalable applications. They advocate using React components, both parent and child components, within a well-structured directory layout. These components are designed for reuse, following best practices for 2023. CodeWalnut also emphasizes maintaining organized codebases as React projects grow, facilitating the use of utility functions, custom components, and libraries like Redux for state management. CodeWalnut developers create reusable UI components, enhance application structure, and optimize performance, making React development smoother and more effective in the ever-evolving web development landscape.
FAQ
1. What are the key benefits of adopting React architecture patterns in 2023, and how can they enhance my development process?
React architecture patterns provide a structured approach to building applications. They make your codebase organized and scalable as your React app grows. This ensures that you can manage the complexity of your project efficiently. Moreover, it follows best practices for 2023 and encourages the use of reusable components, making your code more maintainable and preventing it from turning into spaghetti code. It also plays nicely with libraries like Redux, offering you a convenient way to manage state across your application.
2. Can you explain the significance of separating your React codebase as your project grows, and how does it relate to building React apps efficiently?
As your React app expands, it's crucial to keep your codebase organized and structured. This not only eases maintenance but also enhances team collaboration. Dividing your code into modules and directories helps you locate specific functionality quickly. It's one of the best practices for 2023 as it prevents your codebase from becoming a monolithic and unmaintainable mess. An organized codebase facilitates the integration of new features, custom input components, and data from APIs, making your application more adaptable as it evolves.
3. When building a React app, how can I make the most of the view layer and ensure my front-end development is efficient and smooth?
React excels at providing a robust view layer. To harness its full potential, create reusable UI components and structure your app's architecture efficiently. By separating your code into parent and child components, you can maintain a clear and organized React structure. This approach also supports codebase scalability, especially when working on app development that doesn't require a complete rewrite. Ultimately, React's architecture uses a component tree without prop drilling, resulting in a more maintainable and efficient front-end development process.
4. How can I leverage libraries such as Redux within my React app, and what are the best practices for using them effectively?
Libraries like Redux are powerful tools for managing state in your React app. To use them effectively, follow best practices for 2023, such as adopting a component-based architecture. Organize your application to facilitate the integration of Redux, ensuring that state management aligns with your application's growing needs. By structuring your code, maintaining a clear parent and child component hierarchy, and separating business logic from UI components, you can smoothly incorporate Redux and harness its capabilities for efficient state management.
5. Can you provide an example of a custom hook and how it can enhance my React app development experience?
Certainly! Custom hooks are an excellent way to encapsulate and reuse logic in your React app. For instance, you can create a custom hook for handling data from an API. This hook abstracts the API calls and state management, making your components cleaner and more focused. Using custom hooks follows best practices for ReactJS as it simplifies your codebase, promotes reusability, and keeps your React app organized and efficient.
6. What's the significance of adhering to the best practices for React architecture, and how can they help me in managing multiple internal projects efficiently?
Adhering to the best practices for React architecture is crucial when managing multiple internal projects. It ensures that your codebase remains organized as your projects grow and evolve. This approach prevents a tangled mess of code and promotes maintainability. By following these best practices, you can efficiently navigate different projects, integrate custom components, and leverage shared resources. It's a valuable strategy for a React developer working on various projects, offering a streamlined development process and consistent code quality.
Get in touch - Our team has developed scalable solutions for enterprises and has a Crunch rating of 4.9⭐.
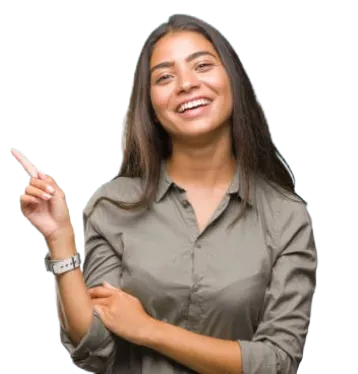
Experience coding prowess firsthand. Choose CodeWalnut to build a prototype within a week and make your choice with confidence.
Accelerate your web app vision with CodeWalnut. In just a week, we'll shape your idea into a polished prototype, powered by Vercel. Ready to make it real? Choose us with confidence!
Dreaming of a powerful web app on Heroku? Let CodeWalnut bring it to life in just one week. Take the leap and trust us to deliver with confidence!