React Design Patterns: Here's What You Need to Know in 2023
React design patterns aren't mere coding conventions; they are the blueprint for building React applications that are both robust and maintainable.
The world of web development is in a constant state of evolution, with new technologies and frameworks emerging at a rapid pace. Amid this ever-changing landscape, React has emerged as a stalwart, providing developers with a robust and flexible library for building user interfaces. React's popularity continues to soar, and as we step into 2023, it remains a cornerstone of modern web development.
However, as applications built with React become increasingly complex and feature-rich, developers are faced with the challenge of maintaining clean, efficient, and scalable code. This is where React design patterns come into play. They serve as guiding principles, providing solutions to common development challenges and best practices for structuring React applications.
Quick Takeaways
- React design patterns promote clean, organized, and modular code, enhancing code quality, reducing bugs, and simplifying onboarding.
- Design patterns offer a scalable architecture, enabling the addition of new features without unnecessary complexity as React applications grow.
- React design patterns encourage code and component reuse, saving time and boosting productivity by reducing redundant work.
Why You Should Follow React Design Patterns?

React design patterns aren't mere coding conventions; they are the blueprint for building React applications that are both robust and maintainable. In this ever-evolving ecosystem, adhering to these patterns offers several compelling reasons why every React developer should make them an integral part of their toolkit in 2023:
1. Code Quality and Maintainability
React design patterns promote clean, organized, and modular code. By following established patterns, developers can create codebases that are easier to read, understand, and maintain. This, in turn, reduces the likelihood of bugs and makes it simpler to onboard new team members.
2. Scalability
As React applications grow in size and complexity, maintaining a clear structure becomes essential. Design patterns provide a scalable architecture that helps developers add new features, components, and functionality without introducing unnecessary complexity or risking codebase instability.
3. Efficiency and Productivity
React design patterns encourage the reuse of components and code, which significantly improves development efficiency. By leveraging pre-defined patterns, developers can save time, reduce redundant work, and boost overall productivity.
4. Team Collaboration
In a collaborative development environment, following design patterns becomes crucial. These patterns serve as a common language for developers, making it easier to collaborate on projects, review code, and understand each other's contributions.
5. Performance Optimization
Certain design patterns are designed to optimize performance. They help minimize unnecessary rendering cycles, optimize state management, and ensure that React applications remain responsive and performant even as they scale.
6. Adherence to Best Practices
React design patterns are based on industry best practices and the collective wisdom of the React community. By following these patterns, developers align their code with established standards, ensuring that their applications are built on a solid foundation.
In this comprehensive guide, we'll explore the most important React design patterns that you need to know in 2023. From component organization to state management, from handling user interactions to code reusability, we'll delve into the patterns and practices that will empower you to build high-quality React applications that meet the demands of modern web development. Whether you're a seasoned React developer or just embarking on your React journey, this guide will equip you with the knowledge and skills to navigate the React landscape with confidence and expertise.
Enabling a Unified Development Platform: Offer a Common Platform to Developers
React, being an open-source library, enjoys a vast and diverse community of developers. This diversity is a strength, but it can also lead to varying coding styles and approaches. React design patterns offer a common language and set of best practices that bridge the gap between developers. When everyone on a project follows the same patterns, it becomes easier to collaborate, understand each other's code, and maintain the project in the long run.
Imagine a scenario where a team of developers is working on a large-scale React application. Without design patterns, each developer might structure their components differently, use different state management techniques, or handle asynchronous data in unique ways. This can quickly turn the codebase into a tangled mess that's challenging to maintain.
By embracing design patterns, teams can establish a shared foundation for development. For example, using the "Presentational and Container Component Pattern" ensures a consistent way of structuring UI components and separating concerns. This commonality simplifies onboarding for new team members, fosters collaboration, and helps prevent common pitfalls associated with inconsistent coding styles.
Promoting React Best Practices: Ensure That React Best Practices Are Applied
React design patterns aren't arbitrary rules imposed on developers. Instead, they are distilled best practices that have emerged from years of experience in building React applications. React's flexibility allows developers to achieve the same goal in multiple ways, but not all ways are created equal.
Design patterns encapsulate the knowledge of the React community about what works well in different scenarios. They provide guidance on how to structure components, manage state, and handle complex interactions effectively. By following these patterns, developers ensure that their code aligns with the best practices endorsed by the React community.
Let's take the example of "Controlled Components." This design pattern advises developers to treat form components as controlled elements, where their value is controlled by the application's state. This approach aligns with React's declarative nature and makes it easier to manage form data. Without this pattern, developers might resort to less efficient methods, leading to code that's harder to maintain and debug.
In essence, React design patterns act as a compass that keeps developers on the right path, steering them away from common pitfalls and guiding them toward code that's efficient, maintainable, and adherent to React's principles.
Now that we've explored the roles of React design patterns, let's delve into some of the most essential patterns that every React developer should be familiar with.
Component Types in React
Functional vs. Container Components
React applications are built by composing components. Two fundamental types of components you'll encounter are Functional Components and Container Components. Understanding when and how to use each is crucial to structuring your application efficiently.
Functional Components
Functional Components, also known as stateless components, are the simplest form of components in React. They are primarily responsible for presenting data and UI elements to the user. Functional Components receive data through props and return JSX to render UI elements. They don't have their internal state.
Here's an example of a Functional Component:
Functional Components are ideal for representing presentational UI elements. They are easy to test, understand, and reuse.
Container Components
Container Components, on the other hand, are responsible for managing state and behavior. They often wrap Functional Components and provide the data and functions required for rendering. Container Components can have their state, and they interact with APIs, manage data fetching, and handle user interactions.
Here's an example of a Container Component:
Container Components are suitable for managing complex logic and state. They enhance the separation of concerns by isolating data management from UI rendering.
By distinguishing between Functional and Container Components, you can create a clean and maintainable component structure. Functional Components focus on rendering, while Container Components handle data and interactions.
Mastering Compound Components: Unveiling the Power of Compound Components
Compound Components are a design pattern that allows you to build components that work together as a group. They enable the creation of complex UI elements with a simple and intuitive API.
Consider a tabbed interface component where each tab's content is defined within the component itself. With Compound Components, you can create a clean and declarative way of defining these tabs:
Using this pattern, you can create tabbed interfaces like this:
The Compound Components pattern promotes encapsulation and reusability. Each Tab component is a child of the Tabs component, and their relationship is established through the API. This design pattern makes it easy to maintain and extend complex UI components.
Harnessing the Power of Conditional Rendering
Conditional rendering is a fundamental concept in React. It involves displaying different components or content based on certain conditions. React's ability to conditionally render components allows you to create dynamic and interactive user interfaces.
Let's look at some common scenarios for conditional rendering:
Conditional Rendering with if Statements
You can use JavaScript if statements to conditionally render components or elements. For example, you might conditionally display a "Login" button if the user is not authenticated:
Conditional Rendering with Ternary Operator
The ternary operator (? :) provides a concise way to conditionally render content based on a condition. It's especially useful when you want to choose between two components:
Conditional Rendering with Logical && Operator
The && operator can be used for conditional rendering when you want to render a component only if a certain condition is true:
In this example, the <p> element is rendered only if isLoggedIn is true.
Conditional Rendering with switch Statements
For more complex conditional rendering scenarios, you can use JavaScript switch statements. Suppose you have multiple cases that determine what to render based on different conditions:
In this example, the Content component renders different types of content based on the contentType prop.
Conditional rendering is a powerful tool in React that allows you to create dynamic user interfaces that adapt to various scenarios. It's essential to understand the different techniques available for conditional rendering and choose the one that best fits your use case.
Utilizing Render Props: Leveraging Render Props for Flexible Component Composition
Render Props is a versatile design pattern in React that involves passing a function as a prop to a component. This function provides data or behavior to the child component, allowing for greater flexibility and reusability.
Let's explore the concept of Render Props with an example:
In this example, the MouseTracker component uses the Render Props pattern. It receives a function via the render prop, which it calls with the current mouse coordinates. The App component defines how to render the MouseTracker and what to do with the mouse coordinates.
The Render Props pattern promotes code reuse and composition. It allows you to create components that provide specific functionality while letting the parent component decide how to use that functionality. This flexibility makes it a valuable tool for building reusable components in React.
Controlling Components in React: Unraveling Controlled Components
Controlled Components are a pattern used in React for handling form elements, such as input fields and checkboxes. In a controlled component, the value of the form element is controlled by the component's state rather than the DOM.
Here's an example of a controlled input field:
In this example, the ControlledInput component manages the input value in its state and updates the value of the input field accordingly. This ensures that the input's value is always in sync with the component's state.
Controlled Components offer several advantages:
- Single Source of Truth: The component's state serves as the single source of truth for the input's value. This simplifies data management and avoids inconsistencies between the UI and application state.
- Predictable Behavior: With a controlled component, you can predict and control the behavior of the input field. You can enforce constraints, validate input, and implement custom logic easily.
- Easier Testing: Testing controlled components is straightforward because you can set the initial state and simulate user interactions to test various scenarios.
- Integration with React State: Controlled components seamlessly integrate with React's state management. You can incorporate input values into the overall application state and use them for various purposes, such as form submission.
Using controlled components is a recommended practice when working with forms in React. It promotes clean and predictable data flow, making form handling more manageable and less error-prone.
Exploring the World of React Hooks: Understanding the Role of React Hooks
React Hooks, introduced in React 16.8, revolutionized how state and side effects are managed in functional components. They provide a more direct API for interacting with React concepts like state, lifecycle methods, and context in functional components.
Let's explore some essential React Hooks:
useState Hook
The useState Hook allows functional components to manage state. It takes an initial state value and returns an array containing the current state and a function to update it.
In this example, the useState Hook is used to manage the count state, and the increment function updates the count when the button is clicked.
useEffect Hook
The useEffect Hook allows functional components to perform side effects, such as data fetching, DOM manipulation, or subscribing to events. It replaces lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount.
In this example, the useEffect Hook fetches data from an API when the component mounts (similar to componentDidMount) and updates the data state when the data is received.
useContext Hook
The useContext Hook allows functional components to access context values. It simplifies working with React's context API.
In this example, the useContext Hook retrieves the current theme value from the ThemeContext and displays it.
React Hooks have become an integral part of React development, providing functional components with the same capabilities as class components. They offer a more concise and expressive way to manage state and side effects, making React development more approachable and enjoyable.
Elevating Patterns with Higher-Order Components: Unpacking the Higher-Order Component Pattern
The Higher-Order Component (HOC) pattern is a powerful technique in React for code reuse, logic sharing, and component composition. It involves wrapping a component with another component that provides additional props or behavior.
Here's how you can create a simple HOC:
In this example, the withLogger HOC takes a component and returns a new component that logs the props before rendering the wrapped component.
HOCs can be used for a wide range of purposes, such as authentication, data fetching, and state management. They enhance the composability and reusability of components.
Adopting Common React Design Patterns
While we've covered several specific React design patterns, it's essential to note that there are many more patterns and best practices that can benefit your React development journey. Some of these patterns are specialized for particular use cases, such as "Provider Pattern" for state management, "Compound Components" for building reusable UI components, and "Render Props" for flexibility in component composition.
As a React developer in 2023, you should strive to be well-versed in these common React design patterns:
- Presentational and Container Components: Master the art of separating UI components from logic, making your codebase more organized and maintainable.
- Provider Pattern: Understand how to manage application-wide state effectively, simplifying complex state-sharing scenarios.
- Higher-Order Component Pattern: Explore the power of HOCs to address cross-cutting concerns like authentication, logging, and data fetching.
- Compound Components: Learn how to create components that work together seamlessly, enhancing reusability and maintainability.
- Conditional Rendering: Master the techniques for rendering components conditionally based on various criteria, providing dynamic user experiences.
- Render Props: Harness the flexibility of the Render Props pattern for creating composable components with interchangeable behavior.
- Controlled Components: Gain expertise in managing form elements and user input using controlled components.
- React Hooks: Embrace the modern approach to state and side effect management in functional components.
Each of these patterns serves as a valuable tool in your React developer toolkit. Depending on your project's requirements, you'll find that certain patterns are more applicable than others. The key is to understand when and how to apply these patterns effectively to create clean, maintainable, and performant React applications.
Building Interactive Prototypes with UXPin Merge and React Components
In the world of UI/UX design and development, creating interactive prototypes is a crucial step. Prototypes allow designers and developers to visualize and test user interactions, ensuring that the final product meets user expectations. One powerful tool for building interactive prototypes with React components is UXPin Merge.
UXPin Merge is a design and prototyping tool that seamlessly integrates with React. It allows designers to create interactive prototypes using actual React components, ensuring a high degree of fidelity between design and development.
Here's how you can use UXPin Merge to build interactive prototypes with React components:
- Design Components: Start by designing your UI components using UXPin's design editor. You can create components, define their styles, and arrange them on artboards just like you would in any design tool.
- Convert to React Components: The magic happens when you convert your designed components into React components using UXPin Merge. This process involves adding React code and logic to your designs to make them interactive.
- Add Interactions: With Merge, you can add interactions to your React components. You can define states, transitions, and user interactions directly in the UXPin interface. This allows you to create complex user flows and test them interactively.
- Preview and Share: UXPin Merge provides a live preview mode that allows you to test your interactive prototypes. You can view and interact with your React components as if they were part of a real application. You can also share your prototypes with team members or stakeholders for feedback.
- Generate Code: Once you're satisfied with your interactive prototype, you can generate production-ready React code directly from UXPin Merge. This code can be the starting point for your actual React application development.
By using UXPin Merge, designers and developers can work closely together, ensuring a smooth transition from design to development. Designers can create interactive prototypes that closely resemble the final product, and developers can use the generated React code as a foundation for building the real application.
This integration between design and development helps reduce misunderstandings and speed up the development process, ultimately leading to a better user experience.
In conclusion, React design patterns and tools like UXPin Merge play vital roles in creating modern, interactive web applications. By understanding and applying React design patterns effectively and leveraging tools that facilitate collaboration between designers and developers, you can build robust, user-friendly, and visually appealing React applications in 2023 and beyond.
Get in touch - Our team has developed scalable solutions for enterprises and has a Crunch rating of 4.9⭐.
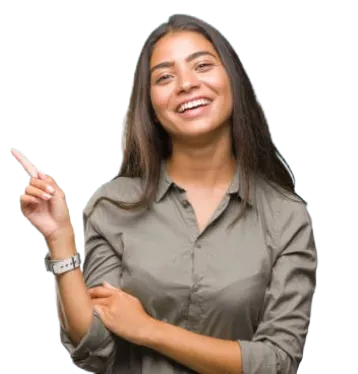
Experience coding prowess firsthand. Choose CodeWalnut to build a prototype within a week and make your choice with confidence.
Accelerate your web app vision with CodeWalnut. In just a week, we'll shape your idea into a polished prototype, powered by Vercel. Ready to make it real? Choose us with confidence!
Dreaming of a powerful web app on Heroku? Let CodeWalnut bring it to life in just one week. Take the leap and trust us to deliver with confidence!